Tokenization
Overview
Tokenization is the process of replacing customer's sensitive card data with a unique identifier, referred to as a token, which can then be saved at the merchant's end to be used later to perform transactions. Tokenization allows merchant to save customer card data in a convenient and secure manner which otherwise requires PCI-DSS compliance certification.
The possible reasons why a merchant might want to save customer card data via tokenization are:
- To improve experience of returning customers so they don't have to enter card details each time they checkout on your website/app. With tokenization in place, it will substitute customer's input of the card number, expiry and name while he/she would only have to input the CVC and OTP to authorize payment. This implementation of tokenization is called CIT - Customer Initiated Transactions.
- To process recurring/unscheduled payments in case the customer has subscribed to a service that requires automatic deduction of funds periodically or against an installment plan, without needing the customer's presence or authorization. In this case, the merchant initiates the transaction from their end to process the payment as per the agreed terms/subscription schedule. This implementation of tokenization is called MIT - Merchant Initiated Transactions.
Token Generation and Pay with Token
The recommended approach for implementing token payments is through the utilization of the "Card/Credentials on file" transaction. In this method, the cardholder provides explicit authorization to the merchant for storing their account information. This information is subsequently replaced with a unique 'token ID'. Token payments enable you to accommodate various billing use-case scenarios for your customers.
3 steps to create a Token and use it for further payments:
- Successful Merchant Authentication
- Successful Token Generation after a successful 3DS transaction
- Use the generated TokenID for receiving further payments.
Authentication
To authenticate the API, you will require the merchant's public key as the username and the API password as the password. Please refer to the section to find your access credentials.
Warning
Your API password is a secret key! It is important to never expose your API password in the front end of your application. Instead, store your API password securely on the server-side, and use a backend proxy to make API requests on behalf of your frontend application. This approach ensures that your API password is never exposed to the client side, reducing the risk of unauthorized access and data breaches.
Token Creation
During initialization of the Hosted Payment Page - while creating the SessionID you should pass "cardOnFile" parameter to true.
- This initial payment must be always performed using 3DS – strong customer authentication and should be Successful.
- Once the initial payment is successful, you will receive a tokenld in the order callback Url.
- Once tokenld is generated it means that Geidea Gateway has saved that payment method for you to use for future payments.
To initiate a Session Creation API call for token creation you will need to provide the following required fields.
Parameter | Description |
---|---|
amount | The total amount value of the payment. This parameter is required, and the format is a double - a number with 2 digits after the decimal point. For example 19.99 |
currency | The currency of the payment. This is a 3-digit alphabetic code, following the ISO 4217 currency code standard. For example: SAR List of available currencies: SAR Saudi RiyalTo enable multicurrency for your account - contact our support team. |
cardOnFile | true |
signature | A parameter to ensure the security and authenticity of API communications. It involves generating a signature using the contents of the API request and secret keys, which is then sent along with the request. |
Please refer to creating signature section here
Usage of the API environment endpoints
Please make sure you use the correct endpoint based on your environment
- KSA Environment: https://api.ksamerchant.geidea.net/
- Egypt Environment: https://api.merchant.geidea.net/
- UAE Environment: https://api.geidea.ae/
Below is an example of creating a session for payment of 10.00 SAR with tokenization parameters.
curl -X POST https://api.merchant.geidea.net/payment-intent/api/v2/direct/session \
-H 'Authorization: Basic <base64 encoded merchant public key and api password>' \
-H 'Content-Type: application/json' \
-d '{
"amount": 10.00,
"currency": "SAR",
"callbackUrl": "https://www.example.com/callback",
"signature": "4Vgy1C4JSLm8o8uxz4Ewj1pv6KbLQ6dj/hu0ExpTWyI=",
"merchantReferenceId": "ABC-123",
"language": "ar"
"cardOnFile": true
}'
<?php
$url = "https://api.merchant.geidea.net/payment-intent/api/v2/direct/session";
$username = "<merchant_public_key>";
$password = "<api_password>";
$data = array(
"amount" => 10.00,
"currency" => "SAR",
"callbackUrl" => "https://example.com/payment-callback",
"merchantReferenceId" => "ORDER-123",
"signature": "4Vgy1C4JSLm8o8uxz4Ewj1pv6KbLQ6dj/hu0ExpTWyI=",
"language" => "ar"
"cardOnFile"=> true
);
$data_json = json_encode($data);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Authorization: Basic ' . base64_encode("$username:$password"),
'Content-Type: application/json',
));
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS,$data_json);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
Below is an example of a successful response you can expect to receive for the request above.
{
"session": {
"id": "9c52785f-f092-4977-7816-08db602e2587",
"amount": 10,
"currency": "SAR",
"callbackUrl": "https://webhook.site/b64f56f2-9423-4368-9aa3-e2de7f7bae33",
"returnUrl": "https://someurl.com",
"expiryDate": "2023-05-31T21:17:00.8733674Z",
"status": "Initiated",
"merchantId": "6876f6bc-f8eb-4253-f160-08d973705ffb",
"merchantPublicKey": "6620c3e2-5088-41a8-8be6-98c003153932",
},
"responseMessage": "Success",
"detailedResponseMessage": "The operation was successful",
"language": "EN",
"responseCode": "000",
"detailedResponseCode": "000"
}
When the transaction is approved if the "cardOnFile: True" parameter is included in the request, the merchant will receive a Token ID in the response. This Token ID can be used by the merchant for subsequent transactions.
Refer to the following instructions when the "detailedStatus" is "Paid." Upon receiving this status, you will obtain the tokenID in the response. You can store this token for future reference, associating it with the customer to facilitate subsequent transactions.
{
"order": {
"orderId": "9e238617-1ae9-469f-02f7-08db602e5619",
"amount": 101,
"tipAmount": 0,
"convenienceFeeAmount": 0,
"totalAmount": 101,
"settleAmount": 101,
"currency": "SAR",
"settleCurrency": "SAR",
"language": "en",
"detailedStatus": "Paid",
"status": "Success",
"threeDSecureId": "98905134-842c-4428-ca9f-08db602e564c",
"merchantId": "e80ece7e-fb2a-4c0b-de94-08d8a29a107b",
"merchantPublicKey": "a087f4ca-9890-407b-9c2f-7630836cc020",
"parentOrderId": null,
"merchantReferenceId": "test-site-5827a75dc9764568ac02457b1bd7b6",
"mcc": null,
"callbackUrl": "https://webhook.site/cd1f0358-4088-4d75-866e-5e5eba5e45fd",
"customerEmail": null,
"billingAddress": {
"countryCode": null,
"street": null,
"city": null,
"postCode": null
},
"shippingAddress": {
"countryCode": null,
"street": null,
"city": null,
"postCode": null
},
"returnUrl": null,
"cardOnFile": true,
"tokenId": "5c430263-0fff-4a1a-f0f7-08db59cbdec9",
"initiatedBy": "Internet",
"agreementId": null,
"agreementType": null,
"paymentOperation": "Pay",
"custom": null,
"paymentIntent": null,
"restrictPaymentMethods": false,
"paymentMethods": null,
"platform": null,
"statementDescriptor": null,
"description": null,
"createCustomer": false,
"setDefaultPaymentMethod": false,
"customerReferenceId": null,
"customerId": null,
"recurrence": null,
"transactions": [
{
"transactionId": "98905134-842c-4428-ca9f-08db602e564c",
"type": "Authentication",
"status": "Success",
"amount": 101,
"currency": "SAR",
"source": "HPP",
"authorizationCode": null,
"rrn": null,
"stan": "0",
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"codes": {
"acquirerCode": null,
"acquirerMessage": null,
"responseCode": "000",
"responseMessage": "Success",
"detailedResponseCode": "000",
"detailedResponseMessage": "The operation was successful"
},
"authenticationDetails": {
"acsEci": "05",
"authenticationToken": "kHyn+7YFi1EUAREAAAAvNUe6Hv8=",
"paResStatus": null,
"veResEnrolled": null,
"xid": "d5f94ec9-f30d-404d-9fb4-d909cc1dac32",
"accountAuthenticationValue": null,
"proofXml": null,
"threeDSecureServerTransactionId": null,
"acsTransactionId": "b806095e-1a86-45cc-bf66-720658b3e003",
"directoryServerId": "A999999999",
"dsTransactionId": "d5f94ec9-f30d-404d-9fb4-d909cc1dac32",
"methodCompleted": false,
"methodSupported": "NOT_SUPPORTED",
"protocolVersion": "2.1.0",
"requestorId": "10065253*MTTEST999999111_MPGS",
"requestorName": "KSA ECR",
"transactionStatus": "Y",
"statusReasonCode": null
},
"postilionDetails": null,
"terminalDetails": null,
"meezaDetails": null,
"bnplDetails": null,
"bankInstallmentDetails": null,
"correlationId": "30178b00-d9ef-418f-af97-674e16bc2ce2",
"parentTransactionId": null,
"paymentAttemptId": "194d374d-a264-44f0-98de-617bfb93b7d9",
"acquirer": {
"additionalResponseData": null,
"batch": null,
"customData": null,
"date": null,
"id": null,
"merchantId": "3000000023",
"settlementDate": null,
"time": null,
"timeZone": null,
"transactionId": null
},
"authorizationResponse": {
"autoExpiry": null,
"avsCode": null,
"cardLevelIndicator": null,
"cardSecurityCodeError": null,
"cardSecurityCodePresenceIndicator": null,
"commercialCard": null,
"commercialCardIndicator": null,
"financialNetworkCode": null,
"financialNetworkDate": null,
"marketSpecificData": null,
"merchantAdviceCode": null,
"paySvcData": null,
"posData": null,
"posEntryMode": null,
"posEntryModeChanged": null,
"processingCode": null,
"responseCode": null,
"date": null,
"responseMessage": null,
"returnAci": null,
"time": null,
"timeZone": null,
"trackQuality": null,
"transactionIdentifier": null,
"transactionIntegrityClass": null,
"validationCode": null,
"vpasResponse": null
},
"madaDetails": null,
"createdDate": "2023-05-31T05:26:46.544697",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:26:55.8477674",
"updatedBy": "PGW"
},
{
"transactionId": "96451299-d3a3-4717-7d64-08db602e760a",
"type": "Pay",
"status": "Success",
"amount": 101,
"currency": "SAR",
"source": "HPP",
"authorizationCode": "100086",
"rrn": "315105100086",
"stan": "100086",
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"codes": {
"acquirerCode": "00",
"acquirerMessage": "Approved",
"responseCode": "000",
"responseMessage": "Success",
"detailedResponseCode": "000",
"detailedResponseMessage": "The operation was successful"
},
"authenticationDetails": null,
"postilionDetails": null,
"terminalDetails": null,
"meezaDetails": null,
"bnplDetails": null,
"bankInstallmentDetails": null,
"correlationId": "3268673d-f8a6-4163-8d89-c6ebb935f5d6",
"parentTransactionId": null,
"paymentAttemptId": "194d374d-a264-44f0-98de-617bfb93b7d9",
"acquirer": {
"additionalResponseData": null,
"batch": 20230531,
"customData": null,
"date": "0531",
"id": "RIYADBANK_S2I",
"merchantId": "3000000023",
"settlementDate": "2023-05-31T00:00:00",
"time": null,
"timeZone": "+0300",
"transactionId": "123456789012345"
},
"authorizationResponse": {
"autoExpiry": null,
"avsCode": null,
"cardLevelIndicator": "88",
"cardSecurityCodeError": null,
"cardSecurityCodePresenceIndicator": null,
"commercialCard": "888",
"commercialCardIndicator": "3",
"financialNetworkCode": null,
"financialNetworkDate": null,
"marketSpecificData": "8",
"merchantAdviceCode": null,
"paySvcData": null,
"posData": "1025100006600",
"posEntryMode": "812",
"posEntryModeChanged": null,
"processingCode": "003000",
"responseCode": "00",
"date": null,
"responseMessage": null,
"returnAci": "8",
"time": null,
"timeZone": null,
"trackQuality": null,
"transactionIdentifier": "123456789012345",
"transactionIntegrityClass": null,
"validationCode": "6789",
"vpasResponse": null
},
"madaDetails": null,
"createdDate": "2023-05-31T05:26:56.7291029",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:26:59.9908664Z",
"updatedBy": "PGW"
}
],
"orderItems": [],
"isTokenPayment": false,
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"totalAuthorizedAmount": 101,
"totalCapturedAmount": 101,
"totalRefundedAmount": 0,
"orderSource": "GeideaGateway",
"paymentBrands": [
"visa"
],
"multiCurrency": {
"authCurrency": "SAR",
"authAmount": 101,
"settleCurrency": "SAR",
"settleAmount": 101,
"exchangeRate": null,
"exchangeFeePercentage": null,
"exchangeFeeAmount": null
},
"isTest": true,
"cashOnDelivery": false,
"amountToCollect": null,
"isDownPayment": false,
"exchangeRate": null,
"exchangeFeePercentage": null,
"exchangeFeeAmount": null,
"deviceId": "14b90417-2522-4479-4b5b-08da6a10e762",
"gatewayDecision": "ContinueToPay",
"subscriptionId": null,
"subscriptionOccurrenceId": null,
"customerPhoneNumber": null,
"customerPhoneCountryCode": null,
"createdDate": "2023-05-31T05:26:46.544697",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:26:50.8563999",
"updatedBy": "PGW"
},
"signature": "C3sjR0O+mcrORORMU4s/MgrvxgJW/2wmROWdoVAQosI="
}
Tokenized Transaction
For Tokenized transactions, the token is used as a reference instead of the original card data. The token is securely transmitted between the parties involved in the transaction, such as the payment gateway, merchant. The token is then used for authorization and payment processing, without the need to handle or store the actual card details.
- The merchant initiates payment on their website using a stored card. The merchant's website retrieves the associated TokenId for the customer and card. Then, the merchant website proceeds with the payment using the provided Token Id.
- The merchant will not receive the complete token, which contains the encrypted card details. Instead, they only receive the TokenId.
- The merchant includes the TokenId, amount, signature and currency in the session request sent to the gateway.
curl -X POST https://api.merchant.geidea.net/payment-intent/api/v2/direct/session \
-H 'Authorization: Basic <base64 encoded merchant public key and api password>' \
-H 'Content-Type: application/json' \
-d '{
"amount": 10.00,
"currency": "SAR",
"callbackUrl": "https://www.example.com/callback",
"merchantReferenceId": "ABC-123",
"signature": "4Vgy1C4JSLm8o8uxz4Ewj1pv6KbLQ6dj/hu0ExpTWyI=",
"language": "ar"
"tokenId": "5c430263-0fff-4a1a-f0f7-08db59cbdec9"
}'
<?php
$url = "https://api.merchant.geidea.net/payment-intent/api/v2/direct/session";
$username = "<merchant_public_key>";
$password = "<api_password>";
$data = array(
"amount" => 10.00,
"currency" => "SAR",
"callbackUrl" => "https://example.com/payment-callback",
"signature": "4Vgy1C4JSLm8o8uxz4Ewj1pv6KbLQ6dj/hu0ExpTWyI=",
"merchantReferenceId" => "ORDER-123",
"language" => "ar"
"tokenId"=>" 5c430263-0fff-4a1a-f0f7-08db59cbdec9"
);
$data_json = json_encode($data);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Authorization: Basic ' . base64_encode("$username:$password"),
'Content-Type: application/json',
));
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS,$data_json);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
Once you have obtained the session ID created along with the token details, pass it to the checkout.html file in order to proceed with the transaction using that specific token.
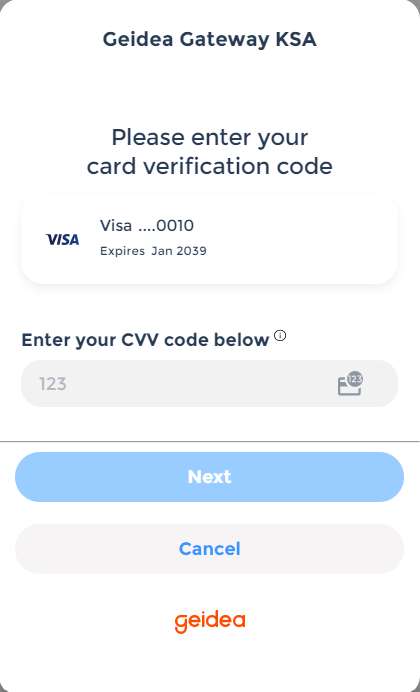
After the transaction is either approved or failed, the merchant will receive the response on the callback URL that they provided during the request creation process.
{
"order": {
"orderId": "7f986e03-798f-4a00-02f9-08db602e5619",
"amount": 100,
"tipAmount": 0,
"convenienceFeeAmount": 0,
"totalAmount": 100,
"settleAmount": 100,
"currency": "SAR",
"settleCurrency": "SAR",
"language": "en",
"detailedStatus": "Paid",
"status": "Success",
"threeDSecureId": "bd9d3cd4-af99-455c-caa1-08db602e564c",
"merchantId": "e80ece7e-fb2a-4c0b-de94-08d8a29a107b",
"merchantPublicKey": "a087f4ca-9890-407b-9c2f-7630836cc020",
"parentOrderId": null,
"merchantReferenceId": "test-site-5827a75dc9764568ac02457b1bd7b6",
"mcc": null,
"callbackUrl": "https://webhook.site/cd1f0358-4088-4d75-866e-5e5eba5e45fd",
"customerEmail": null,
"billingAddress": {
"countryCode": null,
"street": null,
"city": null,
"postCode": null
},
"shippingAddress": {
"countryCode": null,
"street": null,
"city": null,
"postCode": null
},
"returnUrl": null,
"cardOnFile": false,
"tokenId": null,
"initiatedBy": "Internet",
"agreementId": null,
"agreementType": null,
"paymentOperation": "Pay",
"custom": null,
"paymentIntent": null,
"restrictPaymentMethods": false,
"paymentMethods": null,
"platform": null,
"statementDescriptor": null,
"description": null,
"createCustomer": false,
"setDefaultPaymentMethod": false,
"customerReferenceId": null,
"customerId": null,
"recurrence": null,
"transactions": [
{
"transactionId": "bd9d3cd4-af99-455c-caa1-08db602e564c",
"type": "Authentication",
"status": "Success",
"amount": 100,
"currency": "SAR",
"source": "HPP",
"authorizationCode": null,
"rrn": null,
"stan": "0",
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"codes": {
"acquirerCode": null,
"acquirerMessage": null,
"responseCode": "000",
"responseMessage": "Success",
"detailedResponseCode": "000",
"detailedResponseMessage": "The operation was successful"
},
"authenticationDetails": {
"acsEci": "05",
"authenticationToken": "kHyn+7YFi1EUAREAAAAvNUe6Hv8=",
"paResStatus": null,
"veResEnrolled": null,
"xid": "727c4fd7-4b21-4a32-8e80-13e332ea8b46",
"accountAuthenticationValue": null,
"proofXml": null,
"threeDSecureServerTransactionId": null,
"acsTransactionId": "144dd20f-cedd-4e63-919c-900cb768ca85",
"directoryServerId": "A999999999",
"dsTransactionId": "727c4fd7-4b21-4a32-8e80-13e332ea8b46",
"methodCompleted": false,
"methodSupported": "NOT_SUPPORTED",
"protocolVersion": "2.1.0",
"requestorId": "10065253*MTTEST999999111_MPGS",
"requestorName": "KSA ECR",
"transactionStatus": "Y",
"statusReasonCode": null
},
"postilionDetails": null,
"terminalDetails": null,
"meezaDetails": null,
"bnplDetails": null,
"bankInstallmentDetails": null,
"correlationId": "eed9a55b-197a-4eba-96be-dc512799f1ba",
"parentTransactionId": null,
"paymentAttemptId": null,
"acquirer": {
"additionalResponseData": null,
"batch": null,
"customData": null,
"date": null,
"id": null,
"merchantId": "3000000023",
"settlementDate": null,
"time": null,
"timeZone": null,
"transactionId": null
},
"authorizationResponse": {
"autoExpiry": null,
"avsCode": null,
"cardLevelIndicator": null,
"cardSecurityCodeError": null,
"cardSecurityCodePresenceIndicator": null,
"commercialCard": null,
"commercialCardIndicator": null,
"financialNetworkCode": null,
"financialNetworkDate": null,
"marketSpecificData": null,
"merchantAdviceCode": null,
"paySvcData": null,
"posData": null,
"posEntryMode": null,
"posEntryModeChanged": null,
"processingCode": null,
"responseCode": null,
"date": null,
"responseMessage": null,
"returnAci": null,
"time": null,
"timeZone": null,
"trackQuality": null,
"transactionIdentifier": null,
"transactionIntegrityClass": null,
"validationCode": null,
"vpasResponse": null
},
"madaDetails": null,
"createdDate": "2023-05-31T05:30:39.4853845",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:30:46.2010558",
"updatedBy": "PGW"
},
{
"transactionId": "0a59f11d-5fc2-4e97-caa2-08db602e564c",
"type": "Pay",
"status": "Success",
"amount": 100,
"currency": "SAR",
"source": "HPP",
"authorizationCode": "101136",
"rrn": "315105101136",
"stan": "101136",
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"codes": {
"acquirerCode": "00",
"acquirerMessage": "Approved",
"responseCode": "000",
"responseMessage": "Success",
"detailedResponseCode": "000",
"detailedResponseMessage": "The operation was successful"
},
"authenticationDetails": null,
"postilionDetails": null,
"terminalDetails": null,
"meezaDetails": null,
"bnplDetails": null,
"bankInstallmentDetails": null,
"correlationId": "fa6ef30c-4f6d-4cf1-8ec7-d4c3c4a84be2",
"parentTransactionId": null,
"paymentAttemptId": "2858e4dc-1d68-4ab6-91e7-c26c57c9f4ce",
"acquirer": {
"additionalResponseData": null,
"batch": 20230531,
"customData": null,
"date": "0531",
"id": "RIYADBANK_S2I",
"merchantId": "3000000023",
"settlementDate": "2023-05-31T00:00:00",
"time": null,
"timeZone": "+0300",
"transactionId": "123456789012345"
},
"authorizationResponse": {
"autoExpiry": null,
"avsCode": null,
"cardLevelIndicator": "88",
"cardSecurityCodeError": "M",
"cardSecurityCodePresenceIndicator": null,
"commercialCard": "888",
"commercialCardIndicator": "3",
"financialNetworkCode": null,
"financialNetworkDate": null,
"marketSpecificData": "8",
"merchantAdviceCode": null,
"paySvcData": null,
"posData": "1025100006600",
"posEntryMode": "102",
"posEntryModeChanged": null,
"processingCode": "003000",
"responseCode": "00",
"date": null,
"responseMessage": null,
"returnAci": "8",
"time": null,
"timeZone": null,
"trackQuality": null,
"transactionIdentifier": "123456789012345",
"transactionIntegrityClass": null,
"validationCode": "6789",
"vpasResponse": null
},
"madaDetails": null,
"createdDate": "2023-05-31T05:30:47.146083",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:30:48.7494547Z",
"updatedBy": "PGW"
}
],
"orderItems": [],
"isTokenPayment": false,
"paymentMethod": {
"type": "Card",
"brand": "visa",
"cardholderName": "test",
"maskedCardNumber": "444000******0010",
"wallet": null,
"expiryDate": {
"month": 1,
"year": 39
},
"sameBank": false,
"issuingCountry": null,
"fundingType": null,
"issuingBank": null,
"cardCategory": null
},
"totalAuthorizedAmount": 100,
"totalCapturedAmount": 100,
"totalRefundedAmount": 0,
"orderSource": "GeideaGateway",
"paymentBrands": [
"visa"
],
"multiCurrency": {
"authCurrency": "SAR",
"authAmount": 100,
"settleCurrency": "SAR",
"settleAmount": 100,
"exchangeRate": null,
"exchangeFeePercentage": null,
"exchangeFeeAmount": null
},
"isTest": true,
"cashOnDelivery": false,
"amountToCollect": null,
"isDownPayment": false,
"exchangeRate": null,
"exchangeFeePercentage": null,
"exchangeFeeAmount": null,
"deviceId": "14b90417-2522-4479-4b5b-08da6a10e762",
"gatewayDecision": "ContinueToPay",
"subscriptionId": null,
"subscriptionOccurrenceId": null,
"customerPhoneNumber": null,
"customerPhoneCountryCode": null,
"createdDate": "2023-05-31T05:30:39.4853845",
"createdBy": "PGW",
"updatedDate": "2023-05-31T05:30:42.3922011",
"updatedBy": "PGW"
},
"signature": "8JKgXGxLxm3M4RA+Cr0MezepjUSaauVEPivnf+JeFkk="
}
Updated 4 months ago